“Modern C”: Notes on chapter 1 “Getting started”
By Dmitry Kabanov
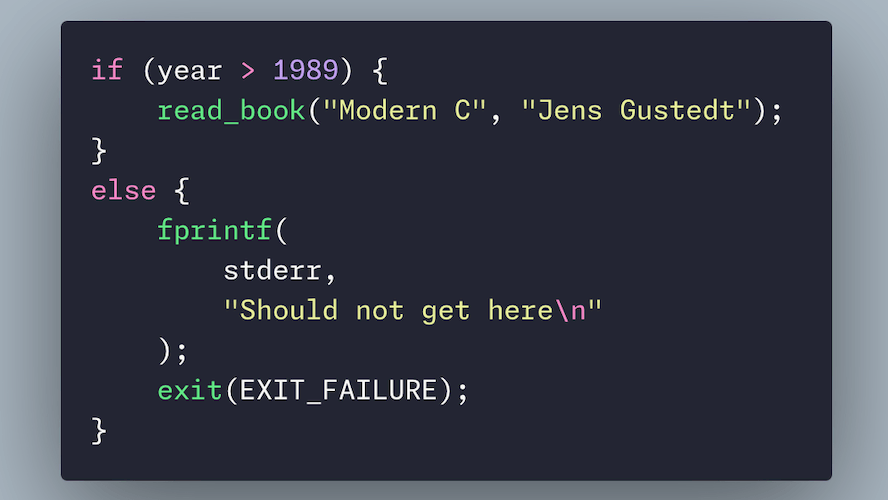
These are my notes taken while reading chapter 1 “Getting Started” from the book “Modern C” by Jens Gustedt. This chapter introduces imperative programming and teaches how to compile and run a C program.
The table of contents for all notes for this book are available in that post.
C is an imperative programming language. A program written in C consists of statements (C term for instructions), such as declaring variables and printing text to screen:
double A[3] = {
[0] = 9.0,
[2] = 3.14,
[1] = 42.42,
};
printf("Element %zu is %g\n", 0, A[0]);
Here we declare an array of double precision floating-point numbers, initialize its elements (by using explicit notation for index and value), and then print a text message about the zeroth (first) element of this array.
The function printf
is probably the most used function in C.
It formats a given string by inserting giving arguments into the string
template.
The things such as %zu
and %g
are format specifiers.
This particular specifiers are for unsigned integer and a double value.
The string literal \n
is used to insert a new line character.
Compiling a program on Linux or macOS can be done using popular free compilers such as GCC and Clang. It is recommended to force at least C99 standard:
gcc -std=c99 -Wall program.c -lm -o program
clang -std=c99 -Wall program.c -lm -o program
In my experience, clang gives slightly more human error messages when things are not quite right. However, as can be seen from the above command examples, they can be used more or less in the same way, at least for simple programs as the command-line arguments are basically the same.
The argument -Wall
tells the compiler to warn about everything that
does not look right to the compiler.
The argument -lm
tell the compiler to link a library that is called simply
m
and includes mathematical functions.
Linking is the process that combines your source code with already compiled
libraries from somebody else (or you - it is quite common to organize
more or less large programs as a collection of libraries).
When the program is compiled successfully, it can be run via
./program
which should print to the screen Element 0 is 9.0
.
The book does not say it, but for simple one-file programs it is also
easy to use Make
.
Make has a built-in (so called implicit) rule for transforming files
like program.c
into an executable file program
.
To use it, one can simply write:
make program
Make
will see that there is the file program.c
on disk and will try
to compile it.
To ensure that the compilation instructions used by Make are the same
as in the above examples, one can write a simple Makefile
like this:
CFLAGS = -std=c99 -Wall
LDLIBS = -lm
It should use by default C compiler cc
, which in macOS is clang
and in Ubuntu gcc
.
Important rule of C programming: A program in C should compile cleanly without warnings.